To follow yesterday progress, I will use the board to fetch the index page of example.org in two scenarios,
- Get an URL served on HTTP
- Get an URL served on HTTPS
There are multiple ways to finish the job, for example, there are different libraries can be used, or, even in the same library, there are still multiple options to implement the same function. So, please notice here, what I mentioned below just a functional way. It’s not the only solution nor the best solution.
Get an URL served on HTTP
The ESP8266 library has really good examples related to HTTPClient, HTTPSClient etc. I copied the source code from the example and made a little change to get an easy output.
#include <WiFiClient.h>
WiFiClient client;
void getURL(String url) {
HTTPClient http;
if (http.begin(client, url)) {
Serial.print("[HTTP] GET ... ");
// start connection and send HTTP header
int httpCode = http.GET();
// httpCode will be negative on error
if (httpCode > 0) {
// HTTP header has been send and Server response header has been handled
Serial.printf("code: %d\n", httpCode);
// file found at server
if (httpCode == HTTP_CODE_OK || httpCode == HTTP_CODE_MOVED_PERMANENTLY) {
String payload = http.getString();
Serial.printf("Payload: %d byte\n", payload.length());
blink(2);
}
} else {
Serial.printf("failed, error: %d %s\n", httpCode, http.errorToString(httpCode).c_str());
blink(5);
}
http.end();
} else {
Serial.printf("[HTTP} Unable to connect\n");
blink(5);
}
}
For a successful case, it will return the length of the payload.
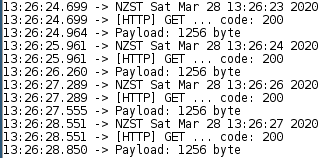
Or if anything unusual happened, it returns the error code and message. Such as,
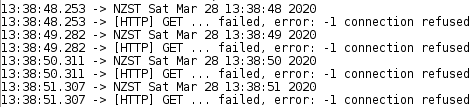
Establish an HTTPS request
To establish an HTTPS request, replace <WiFiClient.h> to <WiFiClientSecure.h> and find a way to validate with the server. In the example “BearSSL_Validation.ino“, most possible ways have been discussed and compared. It’s worth to read. In fetchCertAuthority()
, it shows what will happen with and without NTP synced. I will put the screenshot of the result of the example here.
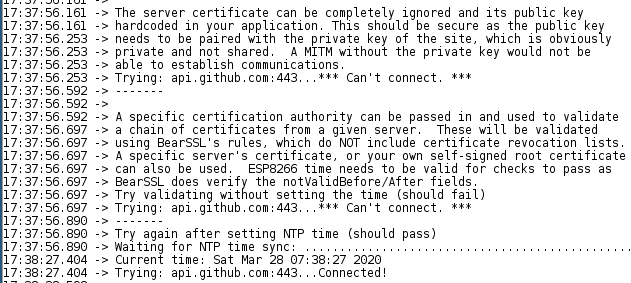
Therefore, the major change would be replacing <WiFiClient.h> to <WiFiClientSecure.h> and using WiFiClientSecure instance instead of WiFiClient. An extra setup is needed.
#include <WiFiClientSecure.h>
WiFiClientSecure client;
static const char digicert[] PROGMEM = R"EOF(
// Something here
)EOF";
X509List* cert;
void setupSecure() {
cert = new X509List(digicert);
client.setTrustAnchors(cert);
}
To get the content of digicert
, I used the following command and copy and paste the content to replace `Something here` above.
echo -n | openssl s_client -connect example.org:443 | sed -ne '/-BEGIN CERTIFICATE-/,/-END CERTIFICATE-/p'
Assembling
Then we can have simple setup()
and loop()
by assembling all these functions together.
void setup() {
Serial.begin(115200);
pinMode(LED_BUILTIN, OUTPUT);
connectWiFi("your-ssid", "your-pass");
setDateTime();
setupSecure();
}
void loop() {
getDateTime();
getURL("https://example.org");
delay(1000);
}
The whole source code has been put on gist. I strongly suggest you give a try to disable and enable line 56 to see how HTTPS affects by NTP.