Most of the times, I want my ESP8266 board to communicate with the server through a securer channel such as MQTT over TLS or HTTPS. However, to verify the certification, system’s date/time has to be in a proper configuration.
Since ESP8266 is designed to have the network, I believe NTP is definitely the first choice and is the easiest one to use. In this post, I will show you how to get date/time and set it by using NTP.
Dummy project
A dummy project is set up for this post. And of course, it can be a part of a real project since the WWW is very important to my most of side projects. The board will do,
- Connect to the WiFi network;
- Use the
GET
method communicate with www.example.com every 5 seconds; - Flash build-in LED twice if 200 was returned by the server;
- Or flash build-in LED 5 times if an error occurred.
Flash LED is the easiest part. But remember to put pinMode(LED_BUILTIN, OUTPUT);
in the very beginning to initialise the output pin.
void blink(int t) {
for(int i = 0; i < t; i ++) {
digitalWrite(LED_BUILTIN, LOW);
delay(500);
digitalWrite(LED_BUILTIN, HIGH);
delay(200);
}
}
Getting date/time
To get the current date/time settings and output to serial, we need to include <time.h> library on the top and use time()
, localtime()
to get the Unix timestamp and convert to the struct to output.
void outputDateTime() {
time_t now = time(nullptr);
struct tm * timeinfo;
timeinfo = localtime(&now);
Serial.printf("%s %s", tzname[0], asctime(timeinfo));
}
Each time, after the board was reset, the system time will start from Unix time 0+28800, which means the local time is UTC 1st Jan 1970 08:00:00 by default.
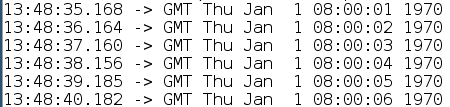
Connect to WiFi
Before to use NTP, the board has to be connected to the Internet. I love ESP8266 just because it doesn’t need a cable, extra adapter board or any hard, brain consuming job to connect it to the Internet. The whole codes here is super easy short and easy to understand. Plenty of documents have detailed the code here. If you have any unsure, comments are welcome.
#include <ESP8266WiFi.h>
void connectWiFi(const char* ssid, const char* password) {
Serial.printf("\nConnecting to %s\n", ssid);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
const char* ip = WiFi.localIP().toString().c_str();
Serial.printf("\nWiFi connected, IP address: %s\n", ip);
}
Setting date/time
On a normal Arduino board, with CurieTime library, the setTime()
function is used for setting the date/time. However, because of the naturality of ESP8266, a function named configTime()
should be used for getting the NTP date/time from the NTP servers and set the time to the board.
There are two declarations of the function,
- void configTime(int timezone_sec, int daylightOffset_sec, const char* server1, const char* server2, const char* server3)
- void configTime(const char* tz, const char* server1, const char* server2, const char* server3)
The one accepts timezone and daylight offset seconds plus 3 NTP servers. Another one accepts the timezone string and 3 NTP servers. I will use the second one and switch to my local time which is Pacific/Auckland. The easiest way to use predefined timezone string is including the <TZ.h> file to the source code.
void setDateTime() {
configTime(TZ_Pacific_Auckland, "pool.ntp.org", "time.nist.gov");
Serial.print("Waiting for NTP time sync: ");
time_t now = time(nullptr);
while (now < 8 * 3600 * 2) {
delay(100);
Serial.print(".");
now = time(nullptr);
}
Serial.println();
struct tm timeinfo;
gmtime_r(&now, &timeinfo);
Serial.printf("%s %s", tzname[0], asctime(&timeinfo));
}
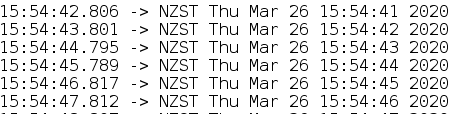
To be continued …
In the next post, I will make a demo to show how to fetch an HTTP page and establish an HTTPS connection with/without NTP synced.
(When I’m writing this post, we are on the first day of lockdown in New Zealand because of COVID-19. I’m planning to recover my writing habit within the stay-at-home prior and start uploading more videos related to programming/3D printing/electronic etc. on Youtube. Be safe, be kind, unite against COVID-19.)
Leave a Reply